Bash (Bourne Again Shell) is a shell command prompt and scripting language in GNU/Linux operating systems. It is the default shell for most Linux distributions.
Conditional statements are vital in any programming language, compiled as well as scripted. They let the user execute a piece of code based on a predefined condition, which is one of the foundations of programming logic. In this article, we will learn how to use the if...else
conditional statement in Bash.
Introduction
The if...else
statement in Bash lets the user manipulate the flow of code based on conditions. User can specify separate code blocks to be executed, only one of which will finally get executed during runtime, based on the corresponding condition which is satisfied.
Note that, more than two conditions can be specified, for which elif
statement can be used. User can give any number of conditions using elif
, and finally a default condition using else
block. Let’s see more about this in the syntax and examples below.
General Syntax
The general syntax for if...else
statement in Bash is:
if <condition>
then
<code block 1>
else
<code block 2>
fi
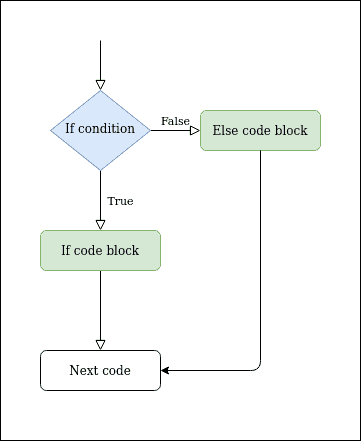
Here, if the <condition>
satisfies, i.e., if it returns 0 (success), then code block 1 is executed. If the condition does not return 0, i.e., it returns a failure status, then the code block 2 is executed. The if...else
block ends with a fi
statement.
For multiple blocks corresponding to multiple conditions, elif
is used:
if <condition 1>
then
<code block 1>
elif <condition 2>
then
<code block 2>
elif <condition 3>
then
<code block 3>
...
...
else
<code block n>
fi
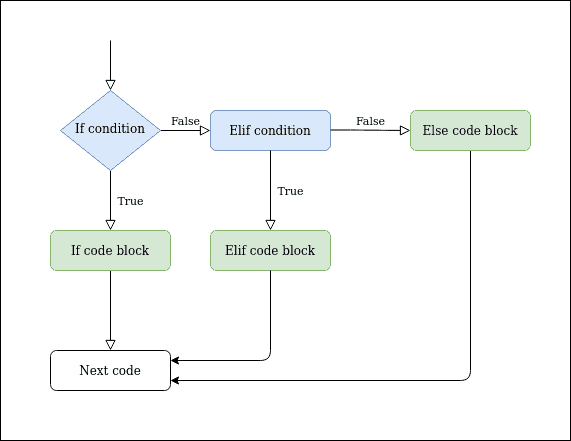
Here, the conditions are checked in order and the code block of the first condition which returns status 0 (success) is executed. Eg. if <condition 1>
returns non zero status (failure), then <condition 2>
is checked. If <condition 2>
returns status 0, <code block 2>
is executed. After this, further conditions are not checked and the code execution continues to the code after the fi
statement.
If none of the conditions return status 0, <code block n>
in the else block is executed. Note that the else block is optional. If no condition is satisfied, as well as no else
block is specified, no conditional code block will run, and code execution will continue to the code after the fi
statement, as shown in the flowgraph below.
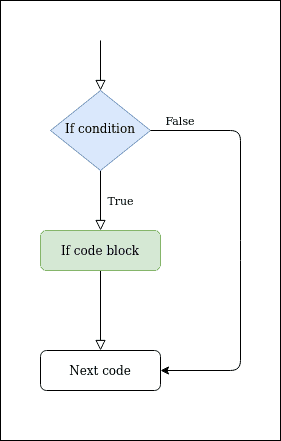
Note that the then
statement is to be used only after if
and elif
statements and not required after the else
statement.
Examples
To execute a code block if a variable has particular value:
x=0
if [ $x -eq 0 ]
then
echo "Value of X is 0"
else
echo "Value of X is not 0"
fi
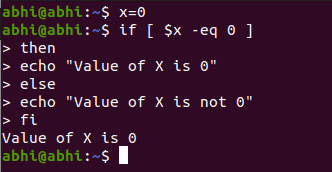
To check for multiple values:
x=2
if [ $x -eq 0 ]
then
echo "Value of X is 0"
elif [ $x -eq 1 ]
then
echo "Value of X is 1"
elif [ $x -eq 2 ]
then
echo "Value of X is 2"
else
echo "Value of X is not 0"
fi
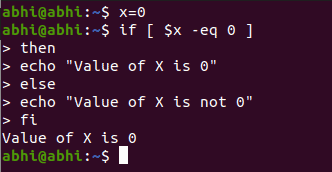
The conditions can be any Linux commands. The corresponding code block will execute if the command runs successfully.
if npm -v
then
echo "NPM present in system"
else
sudo apt install npm
fi
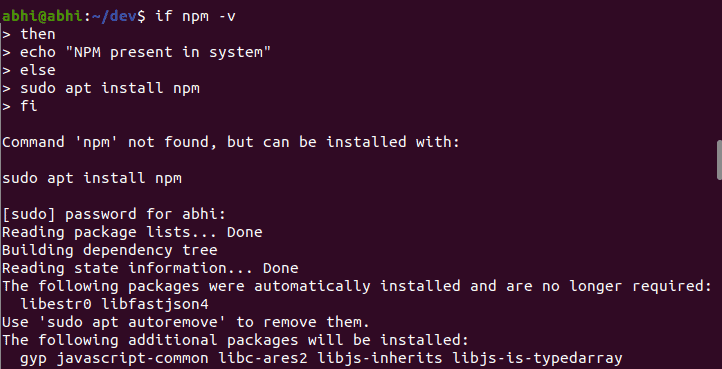
In the example above, since npm
was not installed in the system, the command npm -v
returned a non zero status. Hence, the code execution went into the else
block, where we install npm using apt
package manager. As we can see, it prompted me to enter the password and began installation of npm.
We can also nest another if...else
block inside an if
, else
or elif
block:
x=0
y=1
if [ $x -eq 0 ]
then
echo "X is 0"
if [ $y -eq 1 ]
then
echo "Y is 1"
else
echo "Y is not 1"
fi
else
echo "X is not 0"
fi
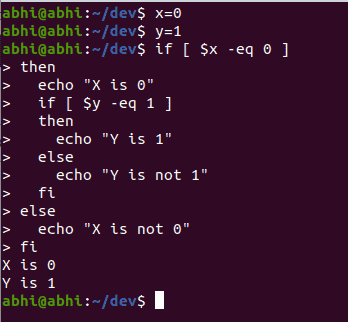
This is useful when a separate set of conditions is to be checked once an initial condition is satisfied.
For example, the following code tries to install a web server software:
if nginx -v
then
echo "NGINX is already installed."
else
if sudo apt install nginx
then
echo "NGINX installation successful."
elif sudo apt install apache2
then
echo "APACHE2 installation successful."
else
echo "Unable to install any web software."
fi
The code first checks if Nginx is already installed in the system. If it is, it just displays a message and exits. If it is not, it tries to install Nginx.
If for some reason, Nginx could not be installed in the system from the package, it tries installing Apache2. If even Apache2 gives error while installing, it exits displaying a message that no software could be installed.
In a similar way, a nested block can be used inside an elif
block as well.
Executing if...else
: Scripts and Command Line
Similar to any Bash code, the if...else
statement can be used in Bash shell directly or from an executable shell script file. Once the Bash interpreter finds an if
, else
or elif
statement, it continues the shell to let the user enter the code block.
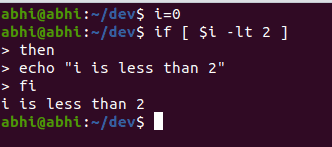
The user can also save this code in a script file and execute the script file.
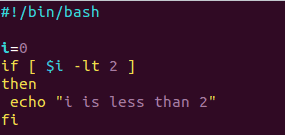
The #!/bin/bash
at the start specifies the interpreter to be used when the file is executed. Although Bash is the most commonly used shell nowadays, some users do prefer shells like zsh, which should be specified in place of bash at the start of this file.
To give execute permissions for this file, run:
chmod +x test.sh
Finally, to execute the file, run:
./test.sh
Conclusion
Similar to any programming language, if...else
statement is a fundamental feature of Bash. Learning its use goes a long way in writing basic as well as advanced scripts.
Member discussion