Bash is used for automating regularly executed commands in Linux. Commands which are meant for operations on files/directories are quite frequently used, and usually, before we execute such a command, we need to check whether the particular file or directory exists. Let us see how to perform this check.
Syntax and Usage
Files
To check if a file exists, use the following syntax:
[ -f <filename> ]
The expression [ -f <filename> ]
returns 0, i.e., success if <filename>
does exist, and if it doesn’t exist it returns a non zero status. Typically, this is used as a condition in the if
statement.
if [ -f <filename> ]
then
<commands>
fi
To negate the returned value, simply precede the -f
with a negation operator (!
).
if [ ! -f <filename> ]
then
<commands>
fi
In this case, the condition will satisfy if the file does not exist, as the returned non zero status (false) will be negated and considered as 0 (true).
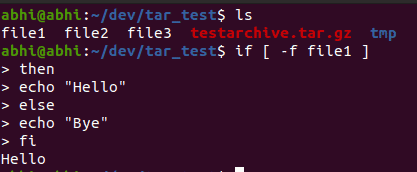
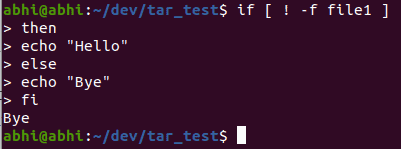
Note that if the file to be checked doesn’t exist in the current directory and exists in some other directory, the full path of the file should be mentioned instead of only the filename.
Directories
Similar syntax as used for checking files is used for checking directories.
[ -d <directory_name> ]
Similar to the statement for files, this statement returns 0, i.e., success, if the directory <directory_name>
exists, and a non zero, i.e. failure status is returned if it does not exist. This is also commonly used in an if
statement condition.
if [ -d <directory_name> ]
then
<commands>
fi
To negate the returned status, precede the -d
with a negation operator (!
).
if [ ! -d <directory_name> ]
then
<commands>
fi
The condition will satisfy (status 0) in this case if the directory does not exist, and will fail (status non zero) if the directory exists.
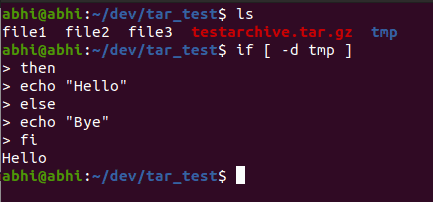
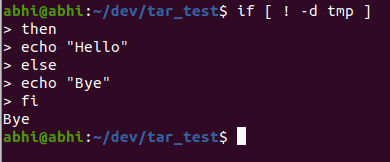
As mentioned before for files, if the directory to be checked exists at another location and not the current directory, the whole directory path needs to be entered instead of just the directory name.
💡 The bracket syntax ( [...]
) used here is actually a Linux command called test
. The options -f
and -d
are the features of this command. For more information, see the manual page of test ( man test
).
Check from a Bash Script
In the above examples, we have used the syntaxes directly on the terminal. The shell whenever it encounters a loop, or a conditional statement (if
in our case), it continues the prompt and lets the user enter the continue the block.
The same syntaxes can be used from inside a Bash script.
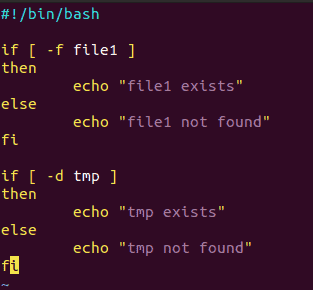
The #!/bin/bash
at the start specifies the interpreter to be used when the file is executed. Although Bash is the most commonly used shell nowadays, some users do prefer shells like zsh
, which should be specified in place of bash at the start of this file.
To give execute permissions for this file, run:
chmod +x test.sh
Finally, to execute the file, run:
./test.sh
Member discussion